Unveiling the Roar of the Crowd: Exploring Sentiment Analysis Techniques with Python
Sentiment analysis, the process of uncovering the emotional tone behind a piece of text, has become a powerful tool for understanding public opinion. By leveraging the capabilities of Python, a versatile programming language, we can unlock valuable insights from social media conversations, product reviews, and other text-based data sources. This article delves into the various methods for performing sentiment analysis using Python, while also exploring its applications in deciphering social media sentiment, particularly on platforms like Twitter, with a specific example of analyzing the emotions surrounding an India-Pakistan cricket match.
Let’s connect
Book a meeting
Sentiment Analysis Techniques in Python’s Arsenal
Python offers a rich ecosystem of libraries and frameworks well-suited for sentiment analysis tasks. In this section, we will delve into several widely used methods:
Lexicon-Based Analysis:
This approach utilizes pre-established dictionaries that include words linked to positive, negative, and neutral sentiments. Libraries like TextBlob and VADER (Valence Aware Dictionary and sEntiment Reasoner) provide pre-defined sentiment lexicons. We can simply calculate the overall sentiment score by counting the occurrences of positive and negative words within the text.
Here’s an example using TextBlob, specifically tailored for analyzing tweets about an India-Pakistan cricket match:
Python
def cricket_sentiment(text):
sentiment = TextBlob(text).sentiment
cricket_sentiment_score = sentiment.polarity
# Add custom logic for cricket specific terms (optional)
for word in cricket_positive_words:
if word in text.lower():
cricket_sentiment_score += 0.1 # Increase score for positive cricket terms
for word in cricket_negative_words:
if word in text.lower():
cricket_sentiment_score -= 0.1 # Decrease score for negative cricket terms
return cricket_sentiment_score
# Analyze a tweet
tweet = “Virat Kohli just smashed a six! #TeamIndia #bleedblue”
sentiment = cricket_sentiment(tweet)print(f”Tweet: {tweet}\nSentiment: {sentiment}”)
Lexicon-based analysis offers a simple and fast approach. However, it can be susceptible to sarcasm and may not capture the nuances of language.
Machine Learning Models:
For more sophisticated sentiment analysis, machine learning models trained on labeled datasets can be employed. Libraries like scikit-learn provide tools for building and deploying such models. We can train a classifier, like Naive Bayes or Support Vector Machines (SVM), on a dataset of cricket-related tweets categorized as positive, negative, or neutral. Once trained, the model can determine the sentiment of new and unseen tweets.
Here’s a glimpse of training a Naive Bayes classifier using scikit-learn, focusing on cricket tweets:
Python
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.naive_bayes import MultinomialNB
# Sample cricket tweets with sentiment labels
tweets = [“India’s bowling attack is unplayable! #champions”,
“Frustrating to see Pakistan lose early wickets. #comebackneeded”,
“This game is tightly contested and keeps everyone on the edge of their seats! #cricket”]
sentiments = [“positive”, “negative”, “neutral”]
# Feature extraction using TfidfVectorizer (considers word importance)
vectorizer = TfidfVectorizer()
features = vectorizer.fit_transform(tweets)
# Training the Naive Bayes model
model = MultinomialNB()
model.fit(features, sentiments)
# Predicting sentiment for a new tweet
new_tweet = “Hoping for a thrilling finish! #IndoPak #CricketFever”
new_features = vectorizer.transform([new_tweet])
predicted_sentiment = model.predict(new_features)[0]
print(f”Predicted sentiment for new tweet: {predicted_sentiment}”)
Machine learning models can achieve higher accuracy than lexicon-based methods, especially when trained on domain-specific data like cricket tweets. However, they require labeled datasets for training, which can be time-consuming to curate.
Deep Learning Models:
Deep learning architectures, such as Long Short-Term Memory (LSTM) networks, offer the most advanced approach to sentiment analysis. LSTMs can capture the sequential nature of language, allowing them to understand the context and sentiment of tweets more effectively. Libraries like TensorFlow and PyTorch provide tools for building and training deep learning models.
Due to the complexity of deep learning, implementing these models requires a strong understanding of deep learning concepts and significant computational resources. However, pre-trained deep learning models for sentiment analysis are becoming increasingly available, making them more accessible to users.
Absolutely, here’s a conclusion summarizing the key takeaways and future possibilities:
In Conclusion
We’ve explored the exciting world of sentiment analysis using Python and its potential to unlock the emotional pulse of a cricket match through social media. We saw how lexicon-based analysis offers a quick and easy approach, while machine learning models with tools like scikit-learn can provide more sophisticated sentiment classification. Deep learning models, although requiring more expertise, hold the potential for the most nuanced understanding of fan emotions.
This journey into sentiment analysis is just the beginning. By expanding our training datasets and exploring evaluation metrics, we can continuously improve the accuracy of our sentiment analysis models. Imagine using these models to analyze real-time tweets during a high-pressure India-Pakistan match, gauging the collective sentiment of millions of fans, and witnessing the emotional rollercoaster unfold word by word. The possibilities are truly thrilling, allowing us to delve deeper into the heart of the game and connect with the unparalleled passion of cricket fans.
Global success stories
Here are some related content that highlight our capability in delivering AI solutions that save costs as well as boost productivity.
Manufacturing 4.0:
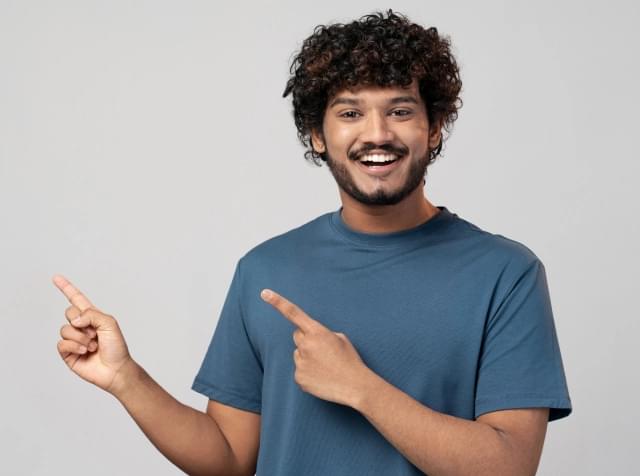